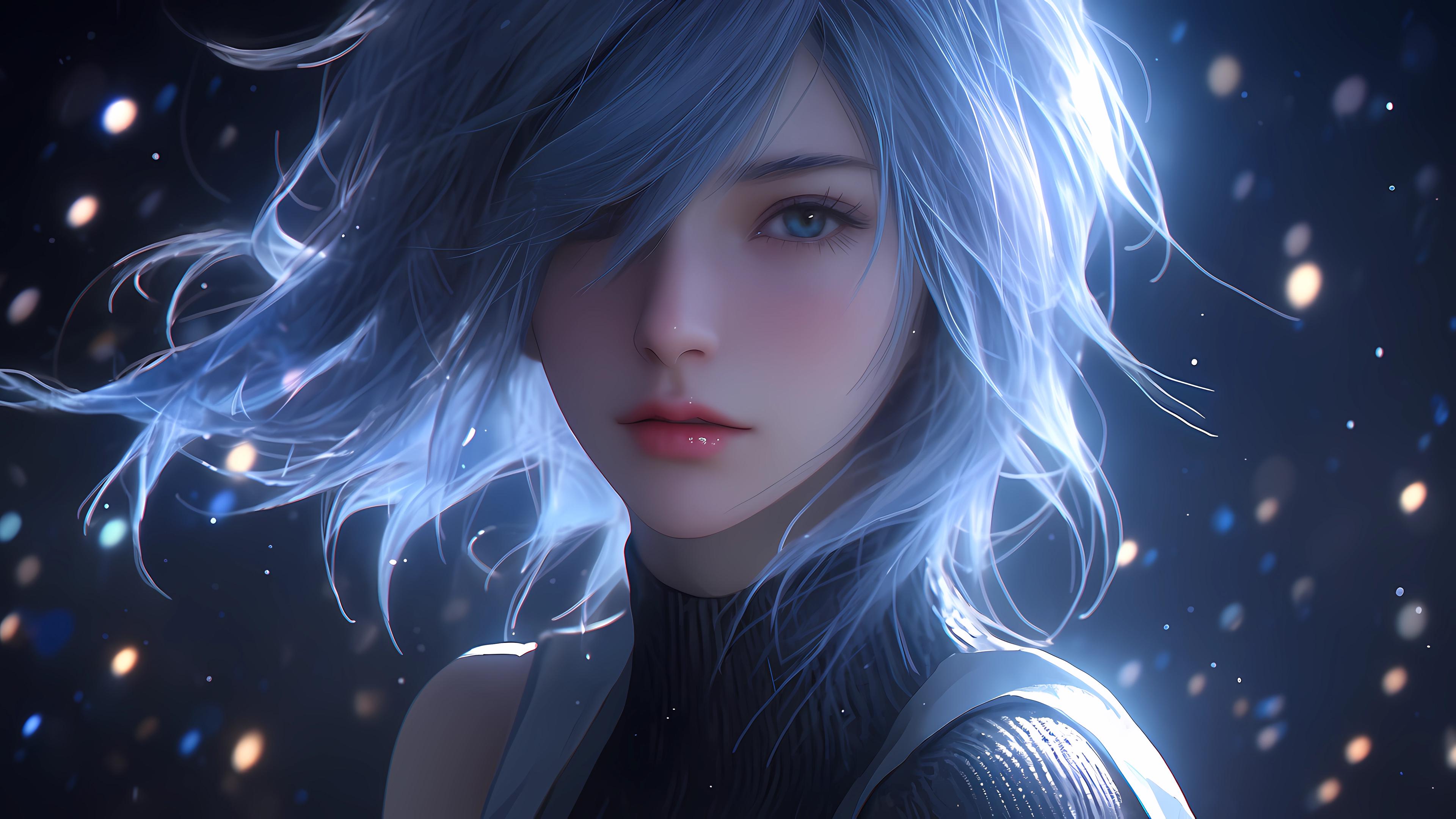
前端如何准确判断数据类型
作者: 夜深了
分类: 前端开发小技巧
发布: 2024-04-11 06:17:37
更新: 2025-03-23 12:47:37
浏览: 109
- 通过typeof 只能判断基础数据类型,对于数组类型,对象,set,map,返回的是object
- 通过 Object.prototype.toString 方法准确判断数据类型
const arr = [1, 2]
const obj = {id: 1, name: "yang"}
const str = "hhhh"
const num = 1000
const sb = Symbol("yyy")
const set = new Set([1,2,3])
const map = new Map()
const func = () => {}
const dataType = (val: any): string => {
let type = Object.prototype.toString.call(val).split(' ')[1].split('').slice(0, -1).join('').toLowerCase()
return type
}
console.log(typeof arr, 'arr') // object arr
console.log(typeof obj, 'obj') // object obj
console.log(typeof str, 'str') // string str
console.log(typeof num, 'num') // number num
console.log(typeof sb, 'sb') // symbol sb
console.log(typeof set, 'set') // object set
console.log(typeof map, 'map') // object map
console.log(typeof func, 'func') // function func
console.log("-----------------------------------")
console.log(dataType(arr), 'arr') // array arr
console.log(dataType(obj), 'obj') // object obj
console.log(dataType(str), 'str') // string str
console.log(dataType(num), 'num') // number num
console.log(dataType(sb), 'sb') // symbol sb
console.log(dataType(set), 'set') // set set
console.log(dataType(map), 'map') // map map
console.log(dataType(func), 'func') // function func
代码如下:
/**
* @description: 判断数据类型
* @param {*} T
* @return {string} 'string','number','object','function' ,'symbol'
*/
const dataType = (val: any): string => {
return Object.prototype.toString.call(val).split(' ')[1].split('').slice(0, -1).join('').toLowerCase()
}
export {
dataType
}